In Windows Forms, Label control is used to display text on the form. It does not interact with user input or handle mouse or keyboard events. Labels are used to provide information to the user within the form such as description, message, or details. These are the key points about labels.
- Display Text or Image: It is mainly used to show the text or image in form.
- Non-Interactive: It is non-interactive and just shows the text not like buttons and textbox.
- Positioning: Labels can be placed anywhere on the form we can use drag and drop and also specify their coordinates using code.
- Auto-Size: Labels can automatically adjust their size and fit according to the content when we set the AutoSize property as true.
Ways To Create Labels in Windows Forms
There are mainly two ways to create labels in the Windows Forms:
- Design Time (Drag and drop)
- Run Time (Custom code)
Design Time ( Drag and drop)
This is the easiest way to create labels in Windows Forms using Visual Studio we just have to open the toolbox and drag and drop the label on the form in the designer and further we can change the appearance of the label using the properties. Follow these steps to create a label.
Step 1: Now locate the project with the name here we are using the default name which is Form1 and it will open a form in the editor that we can further modify.

In the image, we have two files that are open one Design and there is Form1.cs these two play a major role. We use the Form 1.cs file for the custom logic.
Step 2: Now open a Toolbox go to the view > Toolbox or ctrl + alt + x.

Step 3: Choose labels from the common controls in Toolbox as shown below:
And then drag-and-drop in the form:

Step 4. Now open the properties of the label, press right-click on the label and go to properties it will open Solution Explorer now we can change the appearance and behaviour of the label in properties.

Now we can change the appearance and behaviour of the label such as background and text color or font size. These are the changes we made to the label

Similarly, we can create different labels here is the output
Output:

Run Time (Custom Code)
In this method, we are going to modify the Form1.cs file and add custom code modification in C# to change the appearance of the button according to our requirements. Follow these step-by-step processes.
Step 1: Create a label using the Label() constructor provided by the Label class.
// Creating label using Label class
Label mylab = new Label();
Step 2: After creating the Label, set the properties of the Label provided by the Label class.
// Set the text in Label
mylab.Text = “GeeksforGeeks”;
// Set the location of the Label
mylab.Location = new Point(222, 90);
// Set the AutoSize property of the Label control
mylab.AutoSize = true;
// Set the font of the content present in the Label Control
mylab.Font = new Font(“Calibri”, 18);
// Set the foreground color of the Label control
mylab.ForeColor = Color.Green;
// Set the padding in the Label control
mylab.Padding = new Padding(6);
Step 3: And last add this Label control to form using the Add() method.
// Add this label to the form
this.Controls.Add(mylab);
Step 4: Now double-click on the form in Design and it will open the Form.cs file where code is written in C#. Here the program file is Form 1.cs Now write this code in Form1.cs file
Form1.cs file:
C# namespace WinFormsApp1 { public partial class Form1 : Form { public Form1() { InitializeComponent(); } private void Form1_Load(object sender, EventArgs e) { // Creating and setting the label Label mylab = new Label(); mylab.Text = "GeeksforGeeks"; mylab.Location = new Point(222, 90); mylab.AutoSize = true; mylab.Font = new Font("Calibri", 18); mylab.ForeColor = Color.Green; mylab.Padding = new Padding(6); // Adding this control to the form this.Controls.Add(mylab); } } }
Output:
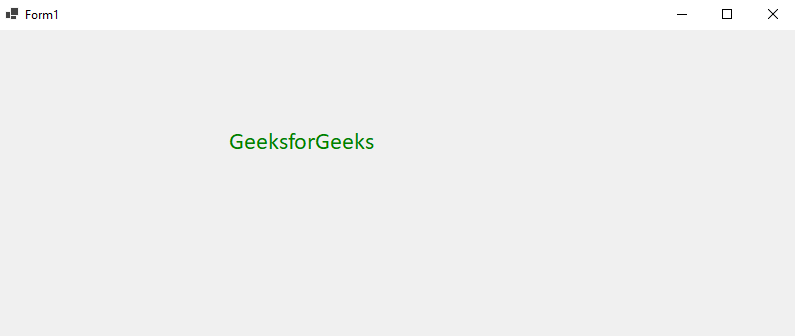
Properties of Label Control
Property | Description |
---|
AutoSize | This property is used to set a value indicating whether the Label control is automatically resized to display its entire contents. |
BackColor | This property is used to set the background colour for the Label control. |
BackgroundImage | This property is used to set the background image for the Label control. |
BorderStyle | This property is used to set the border style for the Label control. |
FlatStyle | This property is used to set the flat style appearance of the label control. |
Font | This property is used to set the font of the text displayed by the Label control. |
FontHeight | This property is used to set the height of the font of the Label control. |
ForeColor | This property is used to set the foreground colour of the Label control. |
Height | This property is used to set the height of the Label control. |
Image | This property is used to set the image that is displayed on a Label. |
Location | This property is used to set the coordinates of the upper-left corner of the Label control relative to the upper-left corner of its form. |
Name | This property is used to set the name of the Label control. |
Padding | This property is used to set padding within the Label control. |
Size | This property is used to set the height and width of the Label control. |
Text | This property is used to set the text associated with this Label control. |
TextAlign | This property is used to set the alignment of text in the label. |
Visible | This property is used to set a value indicating whether the control and all its child controls are displayed. |
Width | This property is used to set the width of the Label control. |
Similar Reads
ref in C#
The ref keyword in C# is used for passing or returning references of values to or from Methods. Basically, it means that any change made to a value that is passed by reference will reflect this change since you are modifying the value at the address and not just the value. It can be implemented in t
4 min read
C# Variables
In C#, variables are containers used to store data values during program execution. So basically, a Variable is a placeholder of the information which can be changed at runtime. And variables allows to Retrieve and Manipulate the stored information. In Brief Defination: When a user enters a new valu
4 min read
while Loop in C#
Looping in a programming language is a way to execute a statement or a set of statements multiple number of times depending on the result of the condition to be evaluated. while loop is an Entry Controlled Loop in C#. The test condition is given in the beginning of the loop and all statements are ex
2 min read
C# Main Thread
In C#, threads are the smallest units of execution that allow parallel execution of code, enabling multiple tasks to run concurrently within a single process. The Thread class in the System.Threading namespace is used to create and manage threads. In C#, the Main method is the entry point of any con
5 min read
C# Tutorial
C# (pronounced "C-sharp") is a modern, versatile, object-oriented programming language developed by Microsoft in 2000 that runs on the .NET Framework. Whether you're creating Windows applications, diving into Unity game development, or working on enterprise solutions, C# is one of the top choices fo
4 min read
C# Literals
In C#, a literal is a fixed value used in a program. These values are directly written into the code and can be used by variables. A literal can be an integer, floating-point number, string, character, boolean, or even null. Example: // Here 100 is a constant/literal.int x = 100; Types of Literals i
5 min read
ulong keyword in C#
Keywords are the words in a language that are used for some internal process or represent some predefined actions. ulong is a keyword that is used to declare a variable which can store an unsigned integer value from the range 0 to 18,446,744,073,709,551,615. It is an alias of System.UInt64. ulong ke
2 min read
C# - if Statement
In C#, if statement is used to indicate which statement will execute according to the value of the given boolean expression. When the value of the boolean expression is true, then the if statement will execute the given then statement, otherwise it will return the control to the next statement after
3 min read
C# StringBuilder
StringBuilder is a Dynamic Object. It doesnât create a new object in the memory but dynamically expands the needed memory to accommodate the modified or new string.A String object is immutable, i.e. a String cannot be changed once created. To avoid string replacing, appending, removing or inserting
4 min read
How to set Text on the Label in C#?
In Windows Forms, Label control is used to display text on the form and it does not take part in user input or in mouse or keyboard events. You are allowed to set the text in the Label control using the Text Property. It makes your label more attractive. You can set this property using two different
3 min read