JSON (JavaScript Object Notation) is a widely-used, lightweight data format for representing structured data. It is used extensively in APIs, configuration files, and data exchange between servers and clients. JSON (JavaScript Object Notation) and XML (eXtensible Markup Language) are popular formats for data representation.
This comprehensive article covers everything we need to know about JSON, including its structure, syntax, applications, and how to work with it in different programming languages.
What is JSON (JavaScript Object Notation)?
JSON stands for JavaScript Object Notation and is a lightweight, text-based data format designed for easy data exchange. JSON is widely used to transmit data between a server and a client as part of a web API response. It is easy to read and write for humans and machines alike, which makes it a preferred choice for data interchange in web applications.
Key Characteristics of JSON
- Text-based: JSON is a simple text format, making it lightweight and easy to transmit.
- Human-readable: It uses key-value pairs, making the structure easy to understand.
- Language-independent: While it is derived from JavaScript, JSON is supported by many programming languages including Python, Java, PHP, and more.
- Data structure: It represents data as objects, arrays, strings, numbers, booleans, and null.
JSON Data Flow: From Server to Client
JSON Data Flow: From Server to Client - JSON TutorialJSON vs XML: A Quick Comparison
When it comes to data formats, JSON and XML are the two most common choices. JSON is generally preferred for web applications due to its smaller size, ease of use, and better performance. Here's a quick comparison:
Feature | JSON | XML |
---|
Readability | Human-readable | Human-readable but more verbose |
Data Size | Smaller and more compact | Larger due to extra markup |
Parsing | Easier to parse in most languages | More complex parsing |
Support | Broad support across languages | Initially JavaScript, but now widely supported |
Use Cases | Web APIs, configuration files, data transfer | Data storage, document formatting |
How JSON Data Flow Works in Web Applications
In a typical web application, JSON (JavaScript Object Notation) is used to transfer data between the server and the client (frontend).
Server Side:
Client Side:
- The JSON string is received as part of an API response (e.g., via an HTTP GET request).
- The client parses this string back into a JavaScript object using JSON.parse().
- The parsed object is then used in the frontend code.
Here’s a JSON string received from the server
const jsonString = '{"name":"Mohit", "age":30}';
It has an object with the properties
To access its properties, we need to parse it into a JavaScript object:
const jsonS = '{"name":"Mohit", "age":30}';
const obj = JSON.parse(jsonS);
let name = obj.name;
let age = obj.age;
console.log(`Name: ${name}, Age: ${age}`);
Output
Name: Mohit, Age: 30
JSON Structure
The basic structure of JSON consists of two primary components:
- Objects: These are enclosed in curly braces {} and contain key-value pairs.
- Arrays: Arrays are ordered lists enclosed in square brackets [].
Objects in JSON
A JSON object is a collection of key-value pairs enclosed in curly braces {}. The key is always a string, and the value can be a variety of data types, including strings, numbers,arrays and even other objects.
Example:
{
"name": "Mohit Kumar",
"age": 30,
"isStudent": false
}
In this example, name
, age
, and isStudent
are keys, and "John Doe"
, 30
, and false
are their respective values.
Arrays in JSON
A JSON array is an ordered collection of values enclosed in square brackets []
. These values can be of any type, including objects, arrays, or primitive data types.
Example:
{
"fruits": ["apple", "banana", "cherry"]
}
Here, fruits
is a key, and the value is an array containing the elements "apple"
, "banana"
, and "cherry"
.
Key JSON Data Types
JSON supports the following data types:
- String: A sequence of characters, e.g.,
"hello"
. - Number: Integer or floating-point numbers, e.g.,
10
, 3.14
. - Boolean: A value representing true or false.
- Array: An ordered list of values.
- Object: A collection of key-value pairs.
- Null: A null value indicating the absence of any value.
How to Work with JSON in JavaScript
In JavaScript, we can easily parse JSON data into a JavaScript object and vice versa using built-in methods like JSON.parse() and JSON.stringify().
Parse JSON to Object
To parse a JSON string into a JavaScript object, use JSON.parse()
.
Example:
let jsonS = '{"name": "Mohit", "age": 30}';
let jsonObj = JSON.parse(jsonS);
console.log(jsonObj.name);
Output
Mohit
Convert Object to JSON
To convert a Javascript object into a JSON string, use JSON.stringify()
Example:
let obj = {name: "Mohit", age: 30};
let jsonS= JSON.stringify(obj);
console.log(jsonS);
Output
{"name": "Mohit", "age" : 30}
How to Work with JSON in Python
Python provides a built-in json
module to work with JSON data. We can use the json.loads()
method to convert a JSON string to a dictionary and json.dumps()
to convert a dictionary to a JSON string.
Parse JSON to Dictionary
import json
json_str = '{"name": "Mohit", "age": 30}'
data = json.loads(json_str)
print(data["name"]) # Output: Mohit
Convert Dictionary to JSON
import json
data = {"name": "Mohit", "age": 30}
json_str = json.dumps(data)
print(json_str) # Output: '{"name": "Mohit", "age": 30}'
Applications of JSON
- APIs: JSON is the most commonly used format for API responses due to its lightweight nature.
- Configuration Files: Many software systems use JSON files for storing configuration data.
- Databases: Some NoSQL databases, like MongoDB, store data in JSON-like formats.
- Data Transfer: JSON is widely used for transferring data between servers and clients, especially in web development.
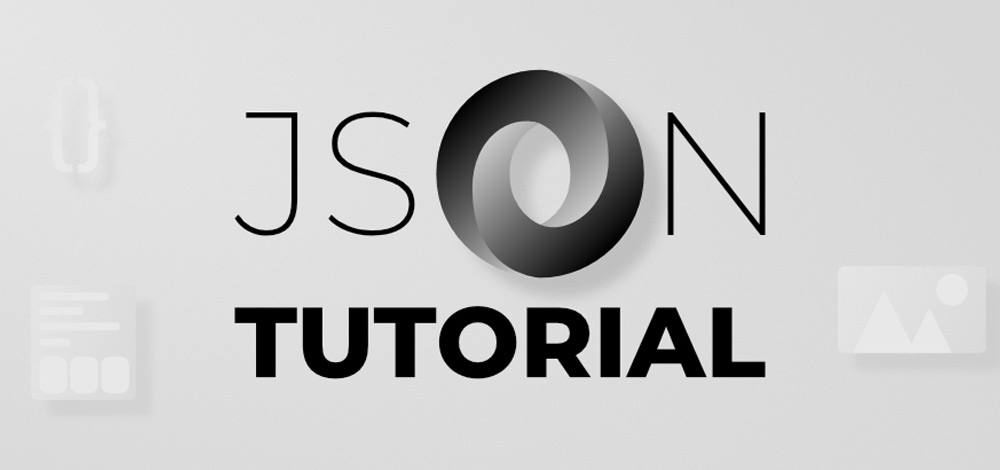
JSON Basics
JSON SetUp
Differences
JSON Methods
Miscellaneous
Important Questions
JSON Interview Questions
Useful JSON Tools
Conclusion
JSON is an essential part of modern web development. Whether we’re working with APIs, configuration files, or data storage, understanding JSON and how to work with it in different programming languages is important for any developer. This article provides a detailed explanation of JSON’s structure, syntax, and usage across multiple platforms. By mastering JSON, we can significantly improve the efficiency and scalability of our web applications.
Similar Reads
JSON Tutorial
JSON (JavaScript Object Notation) is a widely-used, lightweight data format for representing structured data. It is used extensively in APIs, configuration files, and data exchange between servers and clients. JSON (JavaScript Object Notation) and XML (eXtensible Markup Language) are popular formats
6 min read
JSON Introduction
JSON (JavaScript Object Notation) is a lightweight, text-based data format used for representing structured data. It is one of the most widely used formats for data interchange between clients and servers in web applications. JSON has replaced XML as the preferred data format due to its simplicity,
5 min read
JSON Full Form
JSON stands for JavaScript Object Notation. It is a popular data interchange format used in many applications and technology stacks. JSON is easy for both humans and machines to read.It is not tied to any specific programming language and can be combined with C++, Java, and Python.It represents data
1 min read
JSON Data Types
JSON (JavaScript Object Notation) is the most widely used data format for data interchange on the web. JSON is a lightweight text-based, data-interchange format and it is completely language-independent. JSON Data Types JSON supports mainly 6 data types: StringNumberBooleanNullObjectArrayNote: strin
2 min read
JSON Schema
JSON Schema is a content specification language used for validating the structure of a JSON data.It helps you specify the objects and what values are valid inside the object's properties. JSON schema is useful in offering clear, human-readable, and machine-readable documentation. Structure of a JSON
2 min read
JavaScript JSON
JSON (JavaScript Object Notation) is a lightweight data format for storing and exchanging data. It is widely used to send data between a server and a client. JSON is simple, language-independent, and easy to understand. JSON stands for JavaScript Object Notation.It is a lightweight, text-based data
5 min read
JavaScript JSON stringify() Method
The JSON.stringify() method in JavaScript is used to convert JavaScript objects into a JSON string. This method takes a JavaScript object as input and returns a JSON-formatted string representing that object. Syntax: JSON.stringify(value, replacer, space);Parameters: value: It is the value that is t
3 min read
What is JSON text ?
JSON text refers to a lightweight, human-readable format for structuring data using key-value pairs and arrays. It is widely used for data interchange between systems, making it ideal for APIs, configuration files, and real-time communication. In todayâs interconnected digital world, data flows seam
4 min read
Interesting facts about JSON
JSON or JavaScript Object Notation is a lightweight format for transporting and storing data. JSON is used when data is transferred from a server to a web page. This language is also characterized as weakly typed, prototype-based, dynamic, and multi-paradigm. Here are some interesting facts about JS
2 min read