JavaScript localStorage is a feature that lets you store data in your browser using key-value pairs. The data stays saved even after you close the browser, so it can be used again when you open it later. This helps keep track of things like user preferences or state across different sessions.
Syntax
ourStorage = window.localStorage;
The above will return a storage object that can be used to access the current origin’s local storage space.
Key Features of localStorage
- Origin-Bound Storage: Data is stored per domain and is not shared across different origins.
- Persistent Storage: Data remains intact even if the browser is closed or the operating system is rebooted. It will be available until manually cleared.
- Storage Limit: The storage limit for localStorage is 5MB, which is greater than the 4MB limit for cookies.
- No Automatic Transmission: Unlike cookies, localStorage data is not sent with every HTTP request, making it a more efficient option for client-side storage.
Basic Operations with localStorage
localStorage has a simple API that allows you to interact with the browser’s local storage. The basic operations include storing, retrieving, updating, and removing items from the storage.
1. Storing Data in localStorage
To store data in localStorage, use the setItem()
method. This method accepts two arguments:
- The key (a string), which is the identifier for the stored data.
- The value (also a string), which is the data you want to store.
Syntax:
localStorage.setItem('key', 'value');
2. Retrieving Data from localStorage
To retrieve the data you stored, use the getItem()
method. This method takes the key as an argument and returns the associated value. If the key does not exist, it returns null
.
Syntax:
let value = localStorage.getItem('key');
3. Removing Data from localStorage
To remove a specific item from localStorage, use the removeItem()
method. This method accepts the key of the item you want to remove.
Syntax:
localStorage.removeItem('key');
4. Clearing All Data in localStorage
If you want to clear all data stored in localStorage, use the clear()
method. This will remove all key-value pairs stored in the localStorage for the current domain.
Syntax:
localStorage.clear();
5. Checking if a Key Exists in localStorage
You can check if a key exists in localStorage by using getItem()
. If the key doesn’t exist, getItem()
will return null
.
if (localStorage.getItem('username') !== null) {
console.log('Username exists in localStorage');
} else {
console.log('Username does not exist in localStorage');
}
Example: Using localStorage
This example demonstrates using localStorage to store, update, retrieve, and delete key/value pairs in the browser. It shows setting items, updating them, retrieving data by key, checking stored items count, and clearing the storage.
HTML <!DOCTYPE html> <html> <head> <style> div { width: 300px; height: 200px; padding: 20px; border: 2px solid black; background-color: green; color: white; margin: auto; text-align: center; font-size: 1.5rem; } .box { box-sizing: border-box; } </style> </head> <body> <div class="box">GeeksforGeeks</div> <script> // Saving data as key/value pair localStorage.setItem("name", "GeeksforGeeks"); localStorage.setItem("color", "green"); // Updating data localStorage.setItem("name", "GeeksforGeeks(GfG)"); localStorage.setItem("color", "Blue"); // Get the data by key let name = localStorage.getItem("name"); console.log("This is - ", name); let color = localStorage.getItem("color"); console.log("Value of color is - ", color); let key1 = localStorage.key(1); let items = localStorage.length; console.log("Total number of items is ", items); localStorage.removeItem("color"); items = localStorage.length; console.log("After removal, total number of items is ", items); localStorage.clear(); console.log("After clearing all items, total items: ", localStorage.length); </script> </body> </html>
In this Example:
- The code saves the values “GeeksforGeeks” and “green” to localStorage under the keys “name” and “color” respectively.
- It then updates these values by changing the “name” to “GeeksforGeeks(GfG)” and the “color” to “blue”.
- The code retrieves the stored values for “name” and “color” from localStorage and logs them to the console.
- It checks which key is stored at position 1 using localStorage.key(1) and logs it.
- The number of items currently stored in localStorage is calculated using localStorage.length and logged to the console.
- The “color” item is removed from localStorage and the remaining item count is displayed. After that, all stored items are cleared using localStorage.clear().
Output:
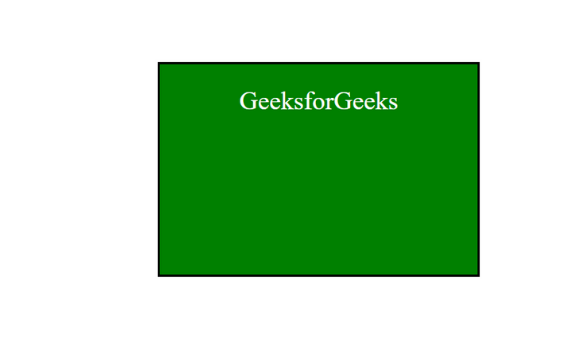
Now, follow the below given steps for performing the basic operations of localStorage:
- Open your web page in the browser.
- Right-click and select “Inspect” or press Ctrl+Shift+I (Windows) or Cmd+Option+I (Mac).
- Go to the “Application” tab.
- In the left-hand menu, under “Storage,” select “Local Storage.”
- Saving data as key/value pair

Updating data
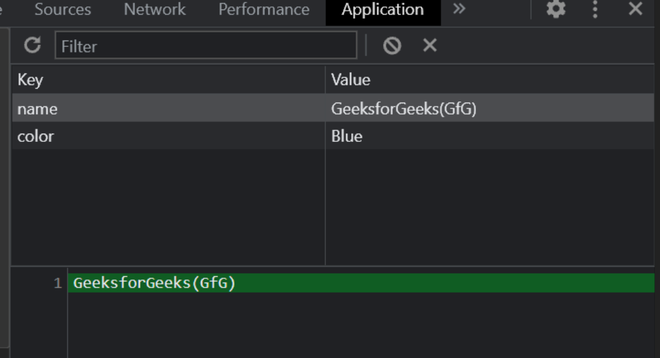
Get data, index of a key, and number of stored items

Remove a key with its value

Delete everything in storage

Storing Non-String Data
localStorage only stores data as strings. If you need to store objects, arrays, or other complex data types, you must convert them into a string format. You can use JSON.stringify()
to convert an object into a JSON string, and JSON.parse()
to convert it back into an object.
Example: Storing and Retrieving an Object
JavaScript // Store an object let user = { name: 'anjali', age: 30 }; localStorage.setItem('user', JSON.stringify(user)); // Retrieve and parse the object let storedUser = JSON.parse(localStorage.getItem('user')); console.log(storedUser.name); // Output: 'anjali' console.log(storedUser.age); // Output: 30
In this example:
- The object
user
is stored as a string using JSON.stringify()
. - When retrieved,
JSON.parse()
is used to convert the string back into an object.
Properties and Methods of localStorage
Method | Description |
---|
setItem(key, value) | Stores a key/value pair |
getItem(key) | Returns the value in front of the key |
key(index) | Gets the key at a given index |
length | Returns the number of stored items (data) |
removeItem(key) | Removes the given key with its value |
clear() | Deletes everything from the storage |
Pros and Cons of Using localStorage
Pros:
- Persistence: Data stored in localStorage remains even after the browser is closed, which is useful for retaining user preferences and session data.
- Simple API: The API for using localStorage is straightforward and easy to use.
- Large Storage Capacity: localStorage allows you to store up to 5MB of data per domain in most browsers.
- No Server Interaction: Data is stored directly in the client’s browser, so it doesn’t require any server-side communication.
Cons:
- Security: Data stored in localStorage is not encrypted, making it vulnerable to cross-site scripting (XSS) attacks. Sensitive data like passwords or tokens should not be stored in localStorage.
- Synchronous: localStorage is a synchronous API, meaning it may block the main thread when storing or retrieving large amounts of data.
- Limited Capacity: The storage capacity (around 5MB) might not be enough for larger datasets.
Browser Support
window.localStorage
is supported in the below given browsers:
Browsers |  |  |  |  |  |
---|
Support | Yes | Yes | Yes | Yes | Yes |
Conclusion
localStorage is an effective tool for storing data persistently within the browser. It provides methods to easily set, retrieve, and manage data, ensuring it remains available across sessions until explicitly cleared. By understanding and utilizing localStorage, developers can enhance the user experience by maintaining state and preferences across browsing sessions.
Similar Reads
JavaScript sessionStorage
JavaScript sessionStorage is a web storage technique that stores data for the duration of a page session. The sessionStorage object lets you store key/value pairs in the browser. It allows setting, retrieving, and managing data that persists only until the browser tab or window is closed, ensuring d
4 min read
JavaScript Local Variables
What are Local Variables in JavaScript?JavaScript local variables are declared inside a block ({} curly braces) or a function. Local variables are accessible inside the block or the function only where they are declared. Local variables with the same name can be used in different functions or blocks
2 min read
JavaScript Memoization
As our systems mature and begin to do more complex calculations, the need for speed grows, and process optimization becomes a need. When we overlook this issue, we end up with applications that take a long time to run and demand a large number of system resources. In this article, we are going to lo
6 min read
JavaScript JSON Parser
JSON (JavaScript Object Notation) is a popular lightweight data exchange format for sending data between a server and a client, or across various systems. JSON data is parsed and interpreted using a software component or library called a JSON parser. Through the JSON parsing process, a JSON string i
3 min read
How to Save Data in Local Storage in JavaScript?
LocalStorage is a client-side web storage mechanism provided by JavaScript, which allows developers to store key-value pairs directly in the user's browser. Unlike cookies, LocalStorage is persistentâdata stored remains even after the browser is closed and reopened, making it ideal for retaining use
2 min read
JavaScript String localeCompare() Method
The string.localeCompare() is an inbuilt method in JavaScript that is used to compare any two elements and returns a positive number if the reference string is lexicographically greater than the compare string and a negative number if the reference string is lexicographically smaller than the compar
4 min read
Local Storage vs Cookies
In JavaScript, there are three primary mechanisms for client-side data storage: cookies, local storage, and session storage. Each has its own use cases and characteristics. Here we will focus on comparing local storage and cookiesâtwo commonly used storage methods in web development. What are Cookie
4 min read
Todo List App Using JavaScript
This To-Do List app helps users manage tasks with features like adding, editing, and deleting tasks. By building it, you'll learn about DOM manipulation, localStorage integration, and basic JavaScript event handling. What We Are Going To CreateWe are creating a Todo application where Users can add,
7 min read
Window Object in JavaScript
In JavaScript, the Window object represents the browser window that contains a DOM document. The Window object offers various properties and methods that enable interaction with the browser environment, including manipulating the document, handling events, managing timers, displaying dialog boxes, a
5 min read
JavaScript History object
The JavaScript History object contains the browser's history. First of all, the window part can be removed from window.history just using the history object alone works fine. The JS history object contains an array of URLs visited by the user. By using the history object, you can load previous, forw
3 min read