Is Node.js entirely based on a single-thread ?
Last Updated : 26 Apr, 2025
Node.js is an open-source and cross-platform runtime environment for executing JavaScript code outside a browser. In this article, we’ll try to dig a little deeper and understand if Node.js is entirely based on a single thread. However, before starting out, let’s clear a few of our basics:
- Process: When a program begins running, it creates a process, which is a program that is now being executed, or a running program. There can be several threads in a process.
- Threads: The smallest group of programmed instructions that may be independently controlled by a scheduler, which is often a component of the operating system, is known as a thread. The main distinction is that whereas processes run in separate memory regions, threads inside a single process use a shared memory space.
- Multiprocessing: The usage of two or more CPUs (processors) within a single computer system is known as multiprocessing. Now that there are numerous processors accessible, numerous processes can run simultaneously.
- Multithreading: Multi-threading is an execution architecture that enables many code blocks (threads) to operate simultaneously within the “context” of a single process.
- Thread Pool: A collection of previously created, unoccupied threads that are waiting to receive work is known as a thread pool. The concept reduces execution delay caused by the frequent generation and destruction of threads for quick activities by keeping a pool of threads.
what actually is meant by a framework being single thread based?
When we say that a framework is single-threaded, that means only one statement is executed at a single time. Whatever is on top of the call stack, gets called in a non-blocking fashion.
Below is the Node.js Server Architecture:
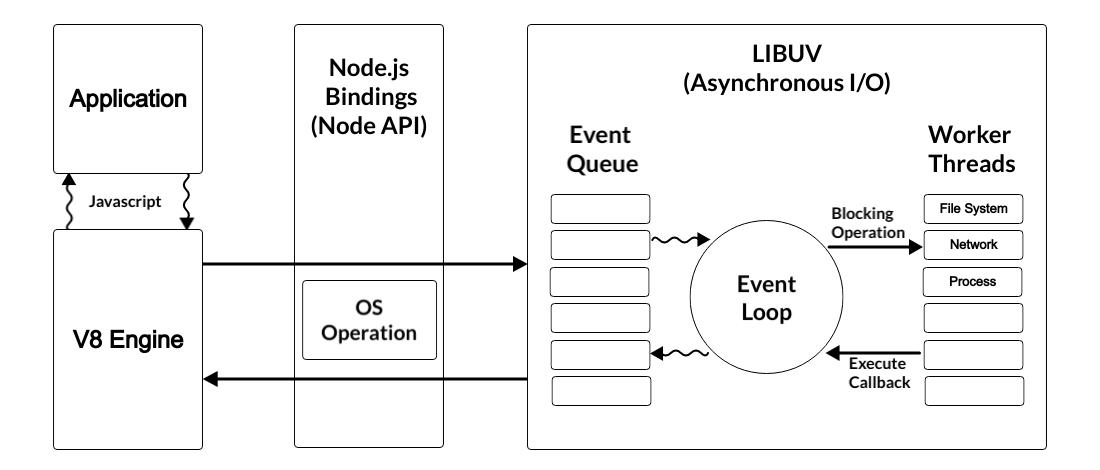
Node.js Architecture
Node.js uses the Single Threaded Event Loop design to manage the numerous concurrent clients. The JavaScript event-based architecture and JavaScript callback mechanism serve as the foundation for the Node.js processing model. A single thread is used to execute a Node.js application, and this thread also houses the event loop. The event loop is at the core of the Node.js processing model. Let us try to understand how the event loop function.
Event Loop: The event loop only performs a single process at a time. This means that it can only execute one function at a time, and since functions can include multiple instructions, the event loop will only execute one instruction at a time. The beauty of the event loop is that it has the ability to “put aside” time-consuming I/O activities so that other instructions can continue to execute without interruption. This is different from running everything on a single thread. This is why, despite the possibility of numerous users simultaneously sending queries to a Node.js API, we receive quick results. Therefore, we may say that Node.js is single-threaded.
How Node.js works as Asynchronous?
However, through the use of worker threads, we can execute instructions as an Asynchronous model. Internally, Node.js makes use of the libuv library, which is a C++ library that manages operating system-related tasks like concurrency, networking, and asynchronous I/O-based operating systems.
This library creates a thread pool of four threads to execute OS-related tasks while making use of all the CPU cores’ processing capacity. It also helps in managing all the other threads. Hence, libuv provides Node.js with multi-threaded capabilities in some cases.
To demonstrate this, let us look at an example:
Example 1: In this example, I have used the pbkdf2 function from the crypto library. I have logged the time it takes from the beginning of the program to run a particular function call.
Javascript
import crypto from 'crypto' ;
const startTime = Date.now();
function logTime() {
crypto.pbkdf2( "a" , "b" , 100000, 512, "sha512" , () => {
console.log( "Hash: " , Date.now() - startTime);
});
}
logTime();
logTime();
logTime();
logTime();
|
Output:
Explanation: As you can see all four function calls, and finish almost at the same time. This is not possible in a single-threaded system. Hence, multiple threads are being used above.
Example 2: In this example, we’ll now for a change, let’s call the function logTime five times:
Javascript
import crypto from 'crypto' ;
const startTime = Date.now();
function logTime() {
crypto.pbkdf2( "a" , "b" , 100000, 512, "sha512" , () => {
console.log( "Hash: " , Date.now() - startTime);
});
}
logTime();
logTime();
logTime();
logTime();
logTime();
|
Output:
Explanation: As you can see four of the function calls execute almost at the same time while the last function call takes a huge time. As the computer has four cores, the first four function calls are executed simultaneously, one function call per core while the last function call is run as soon as any of the cores is free.
Similar Reads
Why Node.js is a Single Threaded Language ?
Node.js is a popular runtime environment that allows developers to build scalable network applications using JavaScript. One of the most distinctive features of Node.js is its single-threaded architecture, which often raises questions among new developers about why it was designed this way. This art
4 min read
What is the Relationship between Node.js and V8 ?
Node.js and V8 are closely related, with V8 being a fundamental component of Node.js that significantly influences its capabilities and performance. Understanding their relationship involves delving into the roles each plays and how they interact within the context of server-side JavaScript executio
10 min read
Why JavaScript is a single-thread language that can be non-blocking ?
The JavaScript within a chrome browser is implemented by a V8 engine. Memory HeapCall Stack Memory Heap: It is used to allocate the memory used by your JavaScript program. Remember memory heap is not the same as the heap data structures, they are totally different. It is the free space inside your O
4 min read
If Node.js is single threaded then how to handles concurrency ?
Node js is an open-source virtual machine that uses javascript as its scripting language. Despite being single-threaded, it is one of the most popular web technologies. The reason why node js is popular despite being single-threaded is the asynchronous nature that makes it possible to handle concurr
4 min read
Difference between Node.js and Ruby on Rails
Before stepping into a new project, the software developing team goes through a severe discussion in order to choose the best language, framework, or methodology for their project. As we are aware, the different technologies have their different pros and cons and similarly the technology which is lo
8 min read
What are the Key Features of Node.js ?
Node.js has gained immense popularity among developers for its ability to handle server-side operations efficiently and effectively. Built on Chrome's V8 JavaScript engine, Node.js is designed to build scalable and high-performance applications. Here, we explore the key features that make Node.js a
5 min read
Why to Use Node.js For Backend Development?
JavaScript is the universal language for building web applications. It is used in frontend (client-side) and backend (server-side) development as well. But the truth that the beauty of the front-end relies on the back-end can't be denied. This is when NodeJS comes into the picture. Node.js is the be
7 min read
How to Use Node.js for Backend Web Development?
In the world of website design nowadays, NodeJS is a supportive tool for making capable backend systems. Whether you are an experienced web designer or just beginning out, NodeJS can upgrade your aptitude and help you in building extraordinary websites. This guide will show you how to use NodeJS to
8 min read
Difference between Node.js and React.js
Node.js and React.js are two powerful technologies widely used in the development of modern web applications. While both are based on JavaScript, they serve entirely different purposes and are used at different stages of the web development process. This article provides a detailed comparison betwee
5 min read
Differences between node.js and Tornado
Node.js and Tornado are both popular choices for building scalable and high-performance web applications and services. However, they are based on different programming languages (JavaScript and Python, respectively) and have distinct design philosophies and features. In this guide, we will explore t
6 min read