How to Make a Chatbot in Python using Chatterbot Module?
Last Updated : 14 Dec, 2020
A ChatBot is basically a computer program that conducts conversation between a user and a computer through auditory or textual methods. It works as a real-world conversational partner.

ChatterBot is a library in python which generates a response to user input. It used a number of machine learning algorithms to generates a variety of responses. It makes it easier for the user to make a chatbot using the chatterbot library for more accurate responses. The design of the chatbot is such that it allows the bot to interact in many languages which include Spanish, German, English, and a lot of regional languages. The Machine Learning Algorithms also make it easier for the bot to improve on its own with the user input.
How To Make a Chatbot in five steps using Python?
We’ll take a step-by-step approach and eventually make our own chatbot.
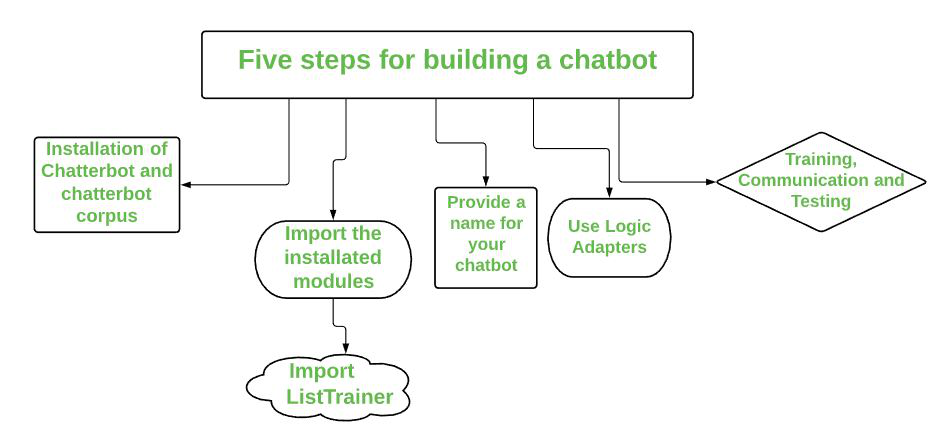
Let’s begin the journey of our own chatbot in the shortest way possible:-
Step 1. Install the Chatterbot and chatterbot_corpus module :
The first and foremost step is to install the chatterbot library. You also need to install the chatterbot_corpus library. Basically, Corpus means a bunch of words. The Chatterbot corpus contains a bunch of data that is included in the chatterbot module. The corpus is used by bots to train themselves.
Run the following pip commands on the terminal for installation:
pip install chatterbot pip install chatterbot_corpus
Step 2. Import the modules
we have to import two classes: ChatBot from chatterbot and ListTrainer from chatterbot.trainers.
ListTrainer: Allows a chatbot to be trained using a list of strings where the list represents a conversation.
Python3
from chatterbot import ChatBot from chatterbot.trainers import ListTrainer |
Step 3. Name our Chatbot:
Now, we will give any name to the chatbot of our choice. Just create a Chatbot object. Here the chatbot is maned as “Bot” just to make it understandable.
Step 4. Use of Logic Adapter:
The Logical Adapter regulates the logic behind the chatterbot that is, it picks responses for any input provided to it. This parameter contains a list of all the logical operators. When more than one logical adapter is put to use, the chatbot will calculate the confidence level, and the response with the highest calculated confidence will be returned as output.
Here we have used two logical adapters:
- BestMatch: The BestMatchAdapter helps it to choose the best match from the list of responses already provided.
- TimeLogicAdapter: The TimeLogicAdapter identifies statements in which a question about the current time is asked. If a matching question is detected, then a response containing the current time is returned.
Python3
chatbot = ChatBot( 'JARVIS' , logic_adapters = [ 'chatterbot.logic.BestMatch' , 'chatterbot.logic.TimeLogicAdapter' ], ) |
Step 5. Training, Communication, and Testing :
For the training process, you will need to pass in a list of statements where the order of each statement is based on its placement in a given conversation. We have to train the bot to improve its performance for this we need to call the train() method by passing a list of sentences. Training ensures that the bot has enough knowledge to get started with specific responses to specific inputs. After training, let’s check its communication skills. And the last step is to do testing
You have to execute the following commands now:
Python3
from chatterbot.trainers import ListTrainer trainer = ListTrainer(bot) trainer.train([ 'Hi' , 'Hello' , 'I need roadmap for Competitive Programming' , 'Just create an account on GFG and start' , 'I have a query.' , 'Please elaborate, your concern' , 'How long it will take to become expert in Coding ?' , 'It usually depends on the amount of practice.' , 'Ok Thanks' , 'No Problem! Have a Good Day!' ]) |
Now let, test the chatbot:
Python3
response = bot.get_response( "Good morning!" ) print (response) |
Output:
Hello
Below is the full implementation:
Python3
from chatterbot import ChatBot from chatterbot.trainers import ListTrainer from chatterbot.trainers import ListTrainer bot = ChatBot( 'Bot' ) trainer = ListTrainer(bot) trainer.train([ 'Hi' , 'Hello' , 'I need roadmap for Competitive Programming' , 'Just create an account on GFG and start' , 'I have a query.' , 'Please elaborate, your concern' , 'How long it will take to become expert in Coding ?' , 'It usually depends on the amount of practice.' , 'Ok Thanks' , 'No Problem! Have a Good Day!' ]) while True : request = input ( 'you :' ) if request = = 'OK' or request = = 'ok' : print ( 'Bot: bye' ) break else : response = bot.get_response(request) print ( 'Bot:' , response) |
Output:
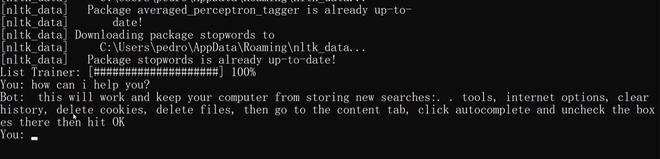
Similar Reads
Chat Bot in Python with ChatterBot Module
Nobody likes to be alone always, but sometimes loneliness could be a better medicine to hunch the thirst for a peaceful environment. Even during such lonely quarantines, we may ignore humans but not humanoids. Yes, if you have guessed this article for a chatbot, then you have cracked it right. We wo
3 min read
How to create modules in Python 3 ?
Modules are simply python code having functions, classes, variables. Any python file with .py extension can be referenced as a module. Although there are some modules available through the python standard library which are installed through python installation, Other modules can be installed using t
4 min read
How to Make API Call Using Python
APIs (Application Programming Interfaces) are an essential part of modern software development, allowing different applications to communicate and share data. Python provides a popular library i.e. requests library that simplifies the process of calling API in Python. In this article, we will see ho
3 min read
Deploy a Chatbot using TensorFlow in Python
In this article, you'll learn how to deploy a Chatbot using Tensorflow. A Chatbot is basically a bot (a program) that talks and responds to various questions just like a human would. We'll be using a number of Python modules to do this. This article is divided into two sections: First, we'll train
9 min read
How to make a voice assistant for E-mail in Python?
As we know, emails are very important for communication as each professional communication can be done by emails and the best service for sending and receiving mails is as we all know GMAIL. Gmail is a free email service developed by Google. Users can access Gmail on the web and using third-party pr
9 min read
How to Use ChatGPT API in Python?
ChatGPT and its inevitable applications. Day by Day everything around us seems to be getting automated by several AI models using different AI and Machine learning techniques and Chatbot with Python , there are numerous uses of Chat GPT and one of its useful applications we will be discussing today.
6 min read
How to Create a Programming Language using Python?
In this article, we are going to learn how to create your own programming language using SLY(Sly Lex Yacc) and Python. Before we dig deeper into this topic, it is to be noted that this is not a beginner's tutorial and you need to have some knowledge of the prerequisites given below. PrerequisitesRou
7 min read
Send Message on Instagram Using Instabot module in Python
In this article, we are going to see how to send messages on Instagram using the Instabot module in Python. Instagram is a nice platform for chatting but when it comes to sending the same message to all friends this is a really boring and time-consuming task especially if you have a large number of
2 min read
How to Install a Python Module?
A module is simply a file containing Python code. Functions, groups, and variables can all be described in a module. Runnable code can also be used in a module. What is a Python Module?A module can be imported by multiple programs for their application, hence a single code can be used by multiple pr
4 min read
Create a ChatBot with OpenAI and Gradio in Python
Computer programs known as chatbots may mimic human users in communication. They are frequently employed in customer service settings where they may assist clients by responding to their inquiries. The usage of chatbots for entertainment, such as gameplay or storytelling, is also possible. OpenAI Ch
3 min read