How to include a template with parameters in EJS ?
Last Updated : 24 Apr, 2025
EJS (Embedded JavaScript) is a templating language that enables dynamic content generation in HTML files using JavaScript syntax. EJS templates consist of HTML code mixed with JavaScript expressions enclosed within <% %> tags.
We will discuss the following approaches to include template with parameters in EJS
Including Templates in EJS:
EJS provides an include function to include other EJS templates within a main template.
To include a template, use the syntax
<%- include('template_name.ejs') %>
where 'template_name.ejs' is the path to the template file. This allows you to reuse common sections of HTML across multiple pages, enhancing code modularity and maintainability.
Steps to Create Application:
Step 1: Create a project folder using the following command.
mkdir ejs-temp
cd ejs-temp
Step 2: Initialize the node application using the following command.
npm init -y
Step 3: Install the required libraries:
npm install express ejs
Folder Structure:
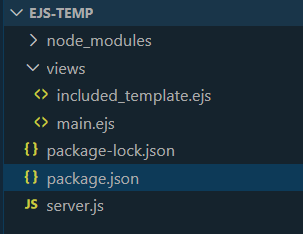
The updated dependencies in package.json file will look like:
"dependencies": {
"ejs": "^3.1.9",
"express": "^4.18.3"
}
Step 4: Create the required folders and fies as seen in the folder structure and Add the following code
JavaScript <!-- included_template.ejs --> <div> <p>Parameter 1: <%= param1 %></p> <p>Parameter 2: <%= param2 %></p> </div>
Approach 1: Using Custom Functions
We define a custom function getParams in server.js to return an object with parameters. We pass this function as a parameter named getParams to the main.ejs template.In main.ejs, we call getParams() within the include statement to dynamically retrieve the parameters and pass them to the included template.
HTML <!-- main.ejs --> <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Main Template</title> </head> <body> <h1>Main Template</h1> <%- include('included_template.ejs', getParams()) %> </body> </html>
JavaScript //server.js const express = require('express'); const ejs = require('ejs'); const app = express(); function getParams() { return { param1: 'Hello', param2: 'World' }; } app.set('view engine', 'ejs'); app.get('/', (req, res) => { res.render('main', { getParams: getParams }); }); app.listen(3000, () => { console.log('Server is running on port 3000'); });
Output:
Approach 2: Using Global Variables
Using global variables, values are assigned to param1 and param2 directly in the server.js file, making them accessible across all EJS templates without needing to pass them explicitly, simplifying the template rendering process.
HTML <!-- main.ejs --> <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Main Template</title> </head> <body> <h1>Main Template</h1> <%- include('included_template.ejs') %> </body> </html>
JavaScript //server.js const express = require('express'); const ejs = require('ejs'); const app = express(); global.param1 = 'Geeks'; global.param2 = 'ForGeeks'; app.set('view engine', 'ejs'); app.get('/', (req, res) => { res.render('main'); }); app.listen(3000, () => { console.log('Server is running on port 3000'); });
Output:
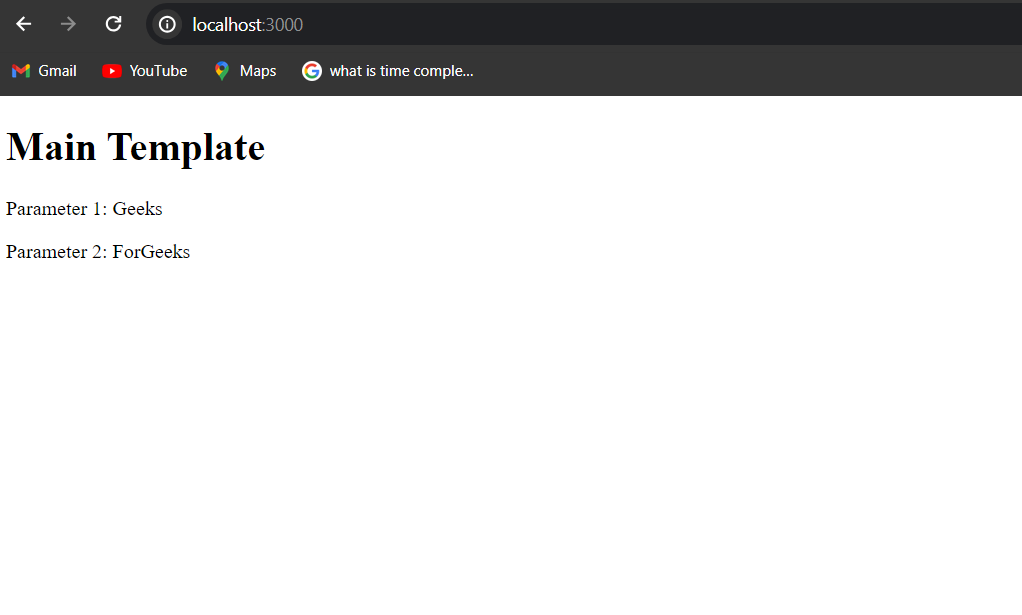
Approach 3: By Passing Data Object
Passing parameters via data object involves creating an object containing the parameters and passing it to the included template's render function. In the main EJS template, you use <%- include('included_template.ejs', data) %> to include the template and pass the data object. Then, in the included template, you can access the parameters using EJS syntax, such as <%= param1 %>.
HTML <!-- main.ejs --> <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Main Template</title> </head> <body> <h1>Main Template</h1> <%- include('included_template.ejs', data) %> </body> </html>
JavaScript //server.js const express = require('express'); const ejs = require('ejs'); const app = express(); app.set('view engine', 'ejs'); app.get('/', (req, res) => { const data = { param1: 'hello', param2: 'geek' }; res.render('main', { data: data }); }); app.listen(3000, () => { console.log('Server is running on port 3000'); });
Output:
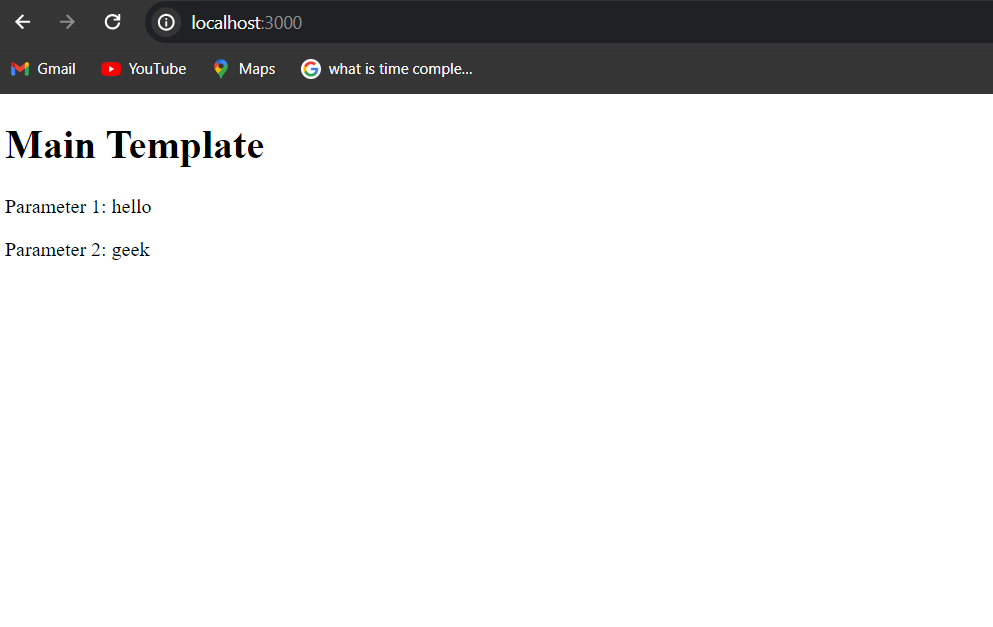
Approach 4: By Passing Parameters Directly
Parameters are passed directly to the included template within the include statement in the main EJS template. This method allows for inline specification of parameter values without the need for intermediate variables. It's a straightforward approach suitable for passing static or predefined values to the included template.
HTML <!-- main.ejs --> <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Main Template</title> </head> <body> <h1>Main Template</h1> <%- include('included_template.ejs', {param1: param1, param2: param2}) %> </body> </html>
JavaScript //server.js const express = require('express'); const ejs = require('ejs'); const app = express(); app.set('view engine', 'ejs'); app.get('/', (req, res) => { res.render('main', { param1: 'Good', param2: 'Evening' }); }); app.listen(3000, () => { console.log('Server is running on port 3000'); });
Output:
Similar Reads
How to include a template with parameters in EJS?
In EJS, we can include templates with parameters for the reuse of modular components in web applications. By passing parameters during the inclusion, we can create interactive templates. In this article, we will see a practical example of including a template with parameters in EJS. Below is the syn
4 min read
How to pass multiple parameter to @Directives in Angular ?
Angular directives are TypeScript classes decorated with the @Directive decorator. These are powerful tools for manipulating the DOM and adding behavior to elements. They can modify the behavior or appearance of DOM elements within an Angular application. Directives can be broadly categorized into t
3 min read
What are the template literals in ES6 ?
Template literals are a new feature that was introduced in ECMAScript6, which offers a simple method for performing string interpolation and multiline string creation. The template literals were called template strings before the introduction of ES6. Starting from ES6 (ECMAScript 6), we have Templat
3 min read
How to Access Request Parameters in Postman?
Request parameters are additional pieces of information sent along with a URL to a server. They provide specific details about the request, influencing the server's response. Parameters typically follow a 'Key=Value' format and are added to the URL in different ways depending on their type. Table of
4 min read
How to use conditional statements with EJS Template Engine ?
Conditional statements in EJS templates allow you to dynamically control the content that is rendered based on specified conditions. Similar to JavaScript, EJS provides a syntax for using conditional logic within your HTML templates. In this article, we will see a practical example of using conditio
3 min read
How to pass query parameters with a routerLink ?
The task is to pass query parameters with a routerLink, for that we can use the property binding concept to reach the goal. Using property binding, we can bind queryParams property and can provide the required details in the object. What is Property Binding? It is a concept where we use square brack
2 min read
How to use template string literal in ECMAScript 6 ?
Template literals are a new feature that was introduced in ECMAScript6, which offers a simple method for performing string interpolation and multiline string creation. The use of the string literal enables the creation of multiline strings free of backslashes, the addition of any word or phrase to t
2 min read
How To Use Query Parameters in NestJS?
NestJS is a popular framework for building scalable server-side applications using Node.js. It is built on top of TypeScript and offers a powerful yet simple way to create and manage APIs. One of the core features of any web framework is handling query parameters, which allow developers to pass data
6 min read
How to Get Query Parameters from URL in Next.js?
In Next.js, getting query parameters from the URL involves extracting key-value pairs from the URL string to access specific data or parameters associated with a web page or application, aiding in dynamic content generation and customization. To get query parameters from the URL in next.js we have m
5 min read
How to use Handlebars to Render HTML Templates ?
Handlebars is a powerful template engine that allows us to create dynamic HTML templates. It helps to render HTML templates easily. One of the advantages of Handlebars is its simplicity, flexibility, and compatibility with various web development frameworks. These are the following methods: Table of
5 min read