How to create a Dictionary App in ReactJS ?
Last Updated : 02 Aug, 2024
In this article, we will be building a very simple Dictionary app with the help of an API. This is a perfect project for beginners as it will teach you how to fetch information from an API and display it and some basics of how React actually works. Also, we will learn about how to use React icons. Let’s begin.
Approach: Our app contains two sections, one for taking the user input and the other is for displaying the data. Whenever a user searches for a word, we store that input in a state and trigger an API call based on the searched keyword parameter. After that when the API call is made, we simply store that API response in another state variable, and then we finally display the information accordingly.
Prerequisites:
The pre-requisites for this project are:
Creating a React application and installing some npm packages:
Step 1: Create a react application by typing the following command in the terminal:
npx create-react-app dictionary-app
Step 2: Now, go to the project folder i.e dictionary-app by running the following command:
cd dictionary-app
Step 3: Let’s install some npm packages required for this project:
npm install axios
npm install react-icons --save
Project Structure: It should look like this:
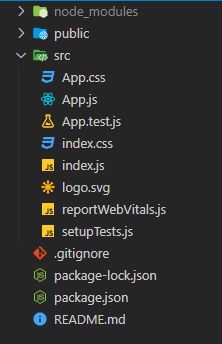
Example: It is the only component of our app that contains all the logic. We will be using a free opensource API (no auth required) called ‘Free Dictionary API’ to fetch all the required data. Our app contains two sections i.e a section for taking the user input and a search button, the other is for displaying the data. Apart from displaying the information that we received, we will also have a speaker button that lets users listen to the phonetics.
Now write down the following code in the App.js file. Here, the App is our default component where we have written our code. Here, the filename is App.js and App.css
JavaScript import { React, useState } from "react"; import Axios from "axios"; import "./App.css"; import { FaSearch } from "react-icons/fa"; import { FcSpeaker } from "react-icons/fc"; function App() { // Setting up the initial states using react hook 'useState' const [data, setData] = useState(""); const [searchWord, setSearchWord] = useState(""); // Function to fetch information on button // click, and set the data accordingly function getMeaning() { Axios.get( `https://api.dictionaryapi.dev/api/v2/entries/en_US/${searchWord}` ).then((response) => { setData(response.data[0]); }); } // Function to play and listen the // phonetics of the searched word function playAudio() { let audio = new Audio(data.phonetics[0].audio); audio.play(); } return ( <div className="App"> <h1>Free Dictionary</h1> <div className="searchBox"> // Taking user input <input type="text" placeholder="Search..." onChange={(e) => { setSearchWord(e.target.value); }} /> <button onClick={() => { getMeaning(); }} > <FaSearch size="20px" /> </button> </div> {data && ( <div className="showResults"> <h2> {data.word}{" "} <button onClick={() => { playAudio(); }} > <FcSpeaker size="26px" /> </button> </h2> <h4>Parts of speech:</h4> <p>{data.meanings[0].partOfSpeech}</p> <h4>Definition:</h4> <p>{data.meanings[0].definitions[0].definition}</p> <h4>Example:</h4> <p>{data.meanings[0].definitions[0].example}</p> </div> )} </div> ); } export default App;
HTML @import url( 'https://fonts.googleapis.com/css2?family=Pacifico&display=swap'); @import url( 'https://fonts.googleapis.com/css2?family=Poppins:ital,wght@0,200;0,400;0,600;0,800;1,300&display=swap'); .App { height: 100vh; width: 100vw; display: flex; flex-direction: column; align-items: center; background-color: #f6f6f6; background-image: linear-gradient(315deg, #f6f6f6 0%, #e9e9e9 74%); font-family:'Poppins', sans-serif; } h1 { text-align: center; font-size: 3em; font-family: 'Pacifico', cursive; color: #4DB33D; padding: 1.5em; } h2{ font-size: 30px; text-decoration: underline; padding-bottom: 20px; } h4{ color: #4DB33D; } input{ width: 400px; height: 38px; font-size: 20px; padding-left: 10px; } .searchBox > button{ background-color: #4DB33D; height: 38px; width: 60px; border: none; color: white; box-shadow: 0px 3px 2px #439e34; cursor: pointer; padding: 0; } .showResults{ width: 500px; padding: 20px; } .showResults > h2 > button{ background: none; border: none; cursor: pointer; }
Step to Run Application: Run the application using the following command from the root directory of the project:
npm start
Output: Now open your browser and go to http://localhost:3000/, you will see the following output:
Similar Reads
BMI Calculator Using React
In this article, we will create a BMI Calculator application using the ReactJS framework. A BMI calculator determines the relationship between a person's height and weight. It provides a numerical value that categorizes the individual as underweight, normal weight, overweight, or obese. Output Previ
3 min read
Create Rock Paper Scissor Game using ReactJS
In this article, we will create Rock, Paper, Scissors game using ReactJS. This project basically implements class components and manages the state accordingly. The player uses a particular option from Rock, Paper, or Scissors and then Computer chooses an option randomly. The logic of scoring and win
6 min read
Create a Form using React JS
Creating a From in React includes the use of JSX elements to build interactive interfaces for user inputs. We will be using HTML elements to create different input fields and functional component with useState to manage states and handle inputs. Prerequisites:Functional ComponentsJavaScript ES6JSXPr
5 min read
Create a Random Joke using React app through API
In this tutorial, we'll make a website that fetches data (joke) from an external API and displays it on the screen. We'll be using React completely to base this website. Each time we reload the page and click the button, a new joke fetched and rendered on the screen by React. As we are using React f
3 min read
Nutrition Meter - Calories Tracker App using React
GeeksforGeeks Nutrition Meter application allows users to input the name of a food item or dish they have consumed, along with details on proteins, calories, fat, carbs, etc. Users can then keep track of their calorie intake and receive a warning message if their calorie limit is exceeded. The logic
9 min read
Currency converter app using ReactJS
In this article, we will be building a very simple currency converter app with the help of an API. Our app contains three sections, one for taking the user input and storing it inside a state variable, a menu where users can change the units of conversion, and finally, a display section where we dis
4 min read
Lap Memory Stopwatch using React
Stopwatch is an application which helps to track time in hours, minutes, seconds, and milliseconds. This application implements all the basic operations of a stopwatch such as start, pause and reset button. It has an additional feature using which we can keep a record of laps which is useful when we
5 min read
Typing Speed Tester using React
In this article, we will create a Typing Speed Tester that provides a random paragraph for the user to type as accurately and quickly as possible within a fixed time limit of one minute. This application also displays the time remaining, counts mistakes calculates the words per minute and characters
9 min read
Number Format Converter using React
In this article, we will create Number Format Converter, that provides various features for users like to conversion between decimal, binary, octal and hexadecimal representations. Using functional components and state management, this program enables users to input a number and perform a range of c
7 min read
Create a Password Validator using ReactJS
Password must be strong so that hackers can not hack them easily. The following example shows how to check the password strength of the user input password in ReactJS. We will use the validator module to achieve this functionality. We will call the isStrongPassword function and pass the conditions a
2 min read