Errors V/s Exceptions In Java
Last Updated : 01 Mar, 2024
In Java, errors and exceptions are both types of throwable objects, but they represent different types of problems that can occur during the execution of a program.
Errors are usually caused by serious problems that are outside the control of the program, such as running out of memory or a system crash. Errors are represented by the Error class and its subclasses. Some common examples of errors in Java include:
- OutOfMemoryError: Thrown when the Java Virtual Machine (JVM) runs out of memory.
- StackOverflowError: Thrown when the call stack overflows due to too many method invocations.
- NoClassDefFoundError: Thrown when a required class cannot be found.
Since errors are generally caused by problems that cannot be recovered from, it’s usually not appropriate for a program to catch errors. Instead, the best course of action is usually to log the error and exit the program.
Exceptions, on the other hand, are used to handle errors that can be recovered from within the program. Exceptions are represented by the Exception class and its subclasses. Some common examples of exceptions in Java include:
- NullPointerException: Thrown when a null reference is accessed.
- IllegalArgumentException: Thrown when an illegal argument is passed to a method.
- IOException: Thrown when an I/O operation fails.
Since exceptions can be caught and handled within a program, it’s common to include code to catch and handle exceptions in Java programs. By handling exceptions, you can provide more informative error messages to users and prevent the program from crashing.
In summary, errors and exceptions represent different types of problems that can occur during program execution. Errors are usually caused by serious problems that cannot be recovered from, while exceptions are used to handle recoverable errors within a program.
In java, both Errors and Exceptions are the subclasses of java.lang.Throwable class. Error refers to an illegal operation performed by the user which results in the abnormal working of the program. Programming errors often remain undetected until the program is compiled or executed. Some of the errors inhibit the program from getting compiled or executed. Thus errors should be removed before compiling and executing. It is of three types:
- Compile-time
- Run-time
- Logical
Whereas exceptions in java refer to an unwanted or unexpected event, which occurs during the execution of a program i.e at run time, that disrupts the normal flow of the program’s instructions.
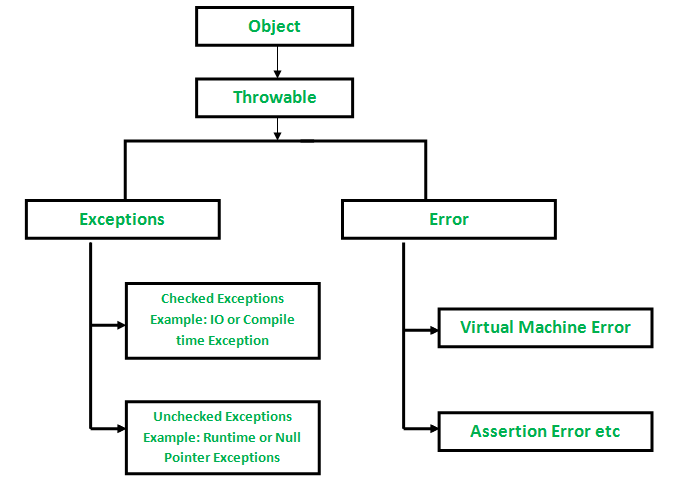
Now let us discuss various types of errors in order to get a better understanding over arrays. As discussed in the header an error indicates serious problems that a reasonable application should not try to catch. Errors are conditions that cannot get recovered by any handling techniques. It surely causes termination of the program abnormally. Errors belong to unchecked type and mostly occur at runtime. Some of the examples of errors are Out of memory errors or System crash errors.
Example 1 Run-time Error
Java
class StackOverflow { public static void test( int i) { if (i == 0 ) return ; else { test(i++); } } } public class GFG { public static void main(String[] args) { StackOverflow.test( 5 ); } } |
Output:
Example 2
Java
class GFG { public static void main(String args[]) { int a = 2 , b = 8 , c = 6 ; if (a > b && a > c) System.out.println(a + " is the largest Number" ); else if (b > a && b > c) System.out.println(b + " is the smallest Number" ); else System.out.println(c + " is the largest Number" ); } } |
Output 8 is the smallest Number
Now let us dwell onto Exceptions which indicates conditions that a reasonable application might want to catch. Exceptions are the conditions that occur at runtime and may cause the termination of the program. But they are recoverable using try, catch and throw keywords. Exceptions are divided into two categories:
Checked exceptions like IOException known to the compiler at compile time while unchecked exceptions like ArrayIndexOutOfBoundException known to the compiler at runtime. It is mostly caused by the program written by the programmer.
Example Exception
Java
class GFG { public static void main(String[] args) { int a = 5 , b = 0 ; try { int c = a / b; } catch (ArithmeticException e) { e.printStackTrace(); } } } |
Output:

Finally now wrapping-off the article by plotting the differences out between them in a tabular format as provided below as follows:
Errors | Exceptions |
Recovering from Error is not possible. | We can recover from exceptions by either using try-catch block or throwing exceptions back to the caller. |
All errors in java are unchecked type. | Exceptions include both checked as well as unchecked type. |
Errors are mostly caused by the environment in which program is running. | Program itself is responsible for causing exceptions. |
Errors can occur at compile time. | Unchecked exceptions occur at runtime whereas checked exceptions occur at compile time |
They are defined in java.lang.Error package. | They are defined in java.lang.Exception package |
Examples : java.lang.StackOverflowError, java.lang.OutOfMemoryError | Examples : Checked Exceptions : SQLException, IOException Unchecked Exceptions : ArrayIndexOutOfBoundException, NullPointerException, ArithmeticException. |
Similar Reads
ArrayStoreException in Java
ArrayStoreException in Java occurs whenever an attempt is made to store the wrong type of object into an array of objects. The ArrayStoreException is a class which extends RuntimeException, which means that it is an exception thrown at the runtime. Class Hierarchy: java.lang.Object ↳ java.lang
2 min read
Chained Exceptions in Java
Chained Exceptions in Java allow associating one exception with another, i.e. one exception describes the cause of another exception. For example, consider a situation in which a method throws an ArithmeticException because of an attempt to divide by zero, but the root cause of the error was an I/O
3 min read
Top 5 Exceptions in Java with Examples
An unexpected unwanted event that disturbs the program's normal execution after getting compiled while running is called an exception. In order to deal with such abrupt execution of the program, exception handling is the expected termination of the program. Illustration: Considering a real-life exa
5 min read
Types of Exception in Java with Examples
Java defines several types of exceptions that relate to its various class libraries. Java also allows users to define their own exceptions. Built-in Exceptions: Built-in exceptions are the exceptions that are available in Java libraries. These exceptions are suitable to explain certain error situati
8 min read
Best Practices to Handle Exceptions in Java
Exception Handling is a critical aspect of Java programming, and following best practices for exception handling becomes even more important at the industry level where software is expected to be highly reliable, maintainable, and scalable. In this article, we will discuss some of the best practices
7 min read
Array Index Out Of Bounds Exception in Java
In Java, ArrayIndexOutOfBoundsException is a Runtime Exception thrown only at runtime. The Java Compiler does not check for this error during the compilation of a program. It occurs when we try to access the element out of the index we are allowed to, i.e. index >= size of the array. Java support
4 min read
Java Exception Handling
Exception handling in Java allows developers to manage runtime errors effectively by using mechanisms like try-catch block, finally block, throwing Exceptions, Custom Exception handling, etc. An Exception is an unwanted or unexpected event that occurs during the execution of a program (i.e., at runt
10 min read
How to Solve Class Cast Exceptions in Java?
An unexcepted, unwanted event that disturbed the normal flow of a program is called Exception. Most of the time exceptions are caused by our program and these are recoverable. Example: If our program requirement is to read data from the remote file locating at the U.S.A. At runtime, if the remote fi
3 min read
User-Defined Custom Exception in Java
In Java, an Exception is an issue (run-time error) that occurs during the execution of a program. When an exception occurs, the program terminates abruptly, and the code beyond the exception never gets executed. Java provides us the facility to create our own exceptions by extending the Exception cl
4 min read
Null Pointer Exception in Java
A NullPointerException in Java is a RuntimeException. In Java, a special null value can be assigned to an object reference. NullPointerException is thrown when a program attempts to use an object reference that has the null value. Example: [GFGTABS] Java // Demonstration of NullPointerException in J
5 min read