Create a QR code generator app using ReactJS
Last Updated : 25 Jul, 2024
In this article, we will create a simple QR Code generator app. A QR code is a two-dimensional barcode that can be read by smartphones. It allows the encoding of more than 4,000 characters in a compact format. QR codes can be used for various purposes, such as displaying text to users, opening URLs, storing contact information in an address book, or sending messages.
Prerequisites:
Approach:
Our app consists of two sections. In the first section, we will collect user inputs such as the text to encode, the size of the QR code, and the background color of the QR code, and store these inputs in state variables. Subsequently, we will construct the necessary API string to fetch the QR code image. In the second section, we will display the generated QR code.
Creating a React application:
Step 1: Create a react application by typing the following command in the terminal.
npx create-react-app qrcode-gen
Step 2: Now, go to the project folder i.e qrcode.gen by running the following command.
cd qrcode-gen
Project Structure:.
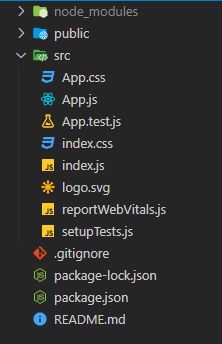
Example: Here App.js is the only default component of our app that contains all the logic. We will be using a free opensource (no auth requires) API called ‘create-qr-code’ to fetch the required QR code image. We will also have a button to download the QR code image.
Now write down the following code in the App.js file.
JavaScript import { useEffect, useState } from 'react'; import './App.css'; function App() { const [temp, setTemp] = useState(""); const [word, setWord] = useState(""); const [size, setSize] = useState(400); const [bgColor, setBgColor] = useState("ffffff"); const [qrCode, setQrCode] = useState(""); // Changing the URL only when the user // changes the input useEffect(() => { setQrCode (`http://api.qrserver.com/v1/create-qr-code/?data=${word}!&size=${size}x${size}&bgcolor=${bgColor}`); }, [word, size, bgColor]); // Updating the input word when user // click on the generate button function handleClick() { setWord(temp); } return ( <div className="App"> <h1>QR Code Generator</h1> <div className="input-box"> <div className="gen"> <input type="text" onChange={ (e) => {setTemp(e.target.value)}} placeholder="Enter text to encode" /> <button className="button" onClick={handleClick}> Generate </button> </div> <div className="extra"> <h5>Background Color:</h5> <input type="color" onChange={(e) => { setBgColor(e.target.value.substring(1)) }} /> <h5>Dimension:</h5> <input type="range" min="200" max="600" value={size} onChange={(e) => {setSize(e.target.value)}} /> </div> </div> <div className="output-box"> <img src={qrCode} alt="" /> <a href={qrCode} download="QRCode"> <button type="button">Download</button> </a> </div> </div> ); } export default App;
Now, let's edit the file named App.css to design our app.
CSS @import url('http://fonts.cdnfonts.com/css/lilly'); .App{ display: flex; flex-direction: column; justify-content: center; align-items: center; gap: 50px; padding-top: 30px; } h1{ font-family: 'Lilly', sans-serif; font-size: 50px; } .gen input{ height: 35px; width: 250px; font-size: 20px; padding-left: 5px; } button{ position: relative; height: 38px; width: 100px; top: -2px; font-size: 18px; border: none; color: whitesmoke; background-color: forestgreen; box-shadow: 2px 2px 5px rgb(74, 182, 74); cursor: pointer; } button:active{ box-shadow: none; } .extra{ padding-top: 20px; display: flex; justify-content: space-around; gap: 10px; } .output-box{ display: flex; flex-direction: column; align-items: center; gap: 40px; }
Step to Run Application: Run the application using the following command from the root directory of the project:
npm start
Output: Now open your browser and go to http://localhost:3000/, you will see the following output: