Commonly Asked Data Structure Interview Questions on Recursion
Last Updated : 24 May, 2025
Recursion is a fundamental concept in computer science, where a function calls itself to solve smaller instances of a problem. Recursion is commonly applied in problems involving trees, graphs, and divide-and-conquer algorithms.
Theoretical Questions for Interviews on Recursion
1. What is recursion?
Recursion is a process where a function calls itself to solve a problem by breaking it down into smaller instances.
For example: calculating the factorial of 4 using recursion
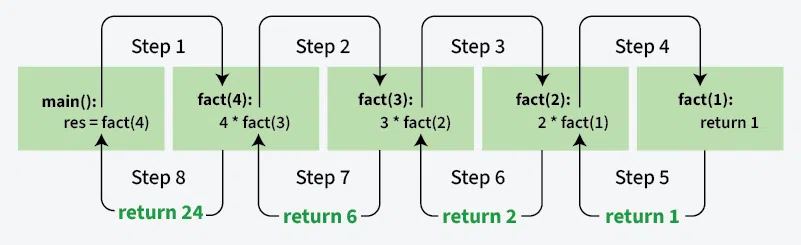
2. What are the base case and recursive case in recursion?
The base case is the condition that stops the recursion, while the recursive case is where the function calls itself with a simpler or smaller input.
3. Can a recursive function have more than one base case?
Yes, a recursive function can have multiple base cases, each handling different stopping conditions for recursion.
4. What is the difference between iteration and recursion?
Iteration uses loops to repeat operations, while recursion involves a function calling itself. Recursion often leads to cleaner solutions but can use more memory due to function calls.
5. What is a stack overflow in recursion?
A stack overflow occurs when the recursion depth exceeds the call stack limit, typically due to missing base case or excessive recursion depth.
5. How memory is allocated to different function calls in recursion?
Recursion uses more memory to store data for each recursive call in the function call stack. Each function call adds a record to the stack, following the LIFO structure. When a function calls itself, memory for the child function is allocated on top of the parent function's memory, with separate copies of local variables for each call. Once the base case is reached, the child function returns its value and is de-allocated, continuing the process.
6. How can recursion be optimized?
Recursion can be optimized using techniques like tail recursion (where the recursive call is the last operation) or memoization (storing results of subproblems to avoid redundant calculations).
7. How does memoization improve the efficiency of recursive functions?
Memoization stores the results of expensive recursive calls so that repeated calls with the same input can return the cached result instead of recalculating it. This reduces time complexity in problems like the Fibonacci sequence and dynamic programming.
8. What is Tail Recursion?
Tail recursion occurs when a function calls itself at the very end of its execution, with no further computation needed after the recursive call returns. In this case, the function’s result is returned directly from the recursive call.
9. Why is Tail Recursion Important?
Tail recursion is beneficial because it can be optimized by compilers, reducing the space complexity of recursive functions. In some languages, it is optimized to run as efficiently as an iterative loop.
10. What is the difference between direct and indirect recursion?
Direct recursion occurs when a function calls itself, while indirect recursion occurs when a function calls another function that eventually calls the original function.
Read more about different types of recusrion Refer, Types of Recursion
11. What is the time complexity of a recursive algorithm?
The time complexity of a recursive algorithm depends on the number of recursive calls and the work done at each step. It's often represented by a recurrence relation:
- For linear recursion: T(n) = T(n-1) + O(1) → O(n)
- For divide-and-conquer: T(n) = 2T(n/2) + O(n) → O(n log n)
12. What is mutual recursion?
Mutual recursion occurs when two or more functions call each other in a cyclic manner. For example: Function A() calls B(), and B() calls A().
13. What is the difference between recursion and backtracking?
Recursion is a general problem-solving technique, while backtracking is a specialized form of recursion where we explore possibilities and revert (backtrack) when a condition is not met.
Top Coding Interview Questions on Recursion
The following list of 50 recursion coding problems covers a range of difficulty levels, from easy to hard, to help candidates prepare for interviews.
Top 50 Problems on Recursion Algorithm asked in SDE Interviews
Similar Reads
Commonly Asked Data Structure Interview Questions on Searching Searching is a fundamental concept in computer science, involving the process of finding a specific element in a collection of data. Efficient searching techniques are crucial for optimizing performance, especially when dealing with large datasets. Top Coding Interview Questions on SearchingThe foll
3 min read
Commonly Asked Data Structure Interview Questions on Sorting Sorting is a fundamental concept in computer science and data structures, often tested in technical interviews. Sorting algorithms are essential for organizing data in a specific order, whether it's ascending or descending. Understanding various sorting techniquesâlike Quick Sort, Merge Sort, Bubble
4 min read
Commonly Asked Data Structure Interview Questions on Stack A stack follows the Last In, First Out (LIFO) principle, meaning the last element added is the first to be removed. Stacks are a fundamental data structure used in many real-world applications, including expression evaluation, function call management, and backtracking algorithms. Theoretical Questi
5 min read
Commonly Asked Data Structure Interview Questions To excel in a Data Structure interview, a strong grasp of fundamental concepts is crucial. Data structures provide efficient ways to store, organize, and manipulate data, making them essential for solving complex problems in software development.Interviewers often test candidates on various data str
6 min read
Commonly Asked Data Structure Interview Questions on Graph A graph is a non-linear data structure that consists of a set of nodes (also known as vertices) connected by edges. Unlike trees, which have a hierarchical structure, graphs can represent more complex relationships, such as social networks, web pages, road maps, and more. This article contains the m
7 min read