Arrays in Golang or Go programming language is much similar to other programming languages. In the program, sometimes we need to store a collection of data of the same type, like a list of student marks. Such type of collection is stored in a program using an Array. An array is a fixed-length sequence that is used to store homogeneous elements in the memory. Due to their fixed length array are not much popular like Slice in Go language. In an array, you are allowed to store zero or more than zero elements in it. The elements of the array are indexed by using the [] index operator with their zero-based position, which means the index of the first element is array[0] and the index of the last element is array[len(array)-1].

Creating and accessing an Array
In Go language, arrays are created in two different ways:
Using var keyword: In Go language, an array is created using the var keyword of a particular type with name, size, and elements. Syntax:
Var array_name[length]Type
Important Points:
In Go language, arrays are mutable, so that you can use array[index] syntax to the left-hand side of the assignment to set the elements of the array at the given index.
Var array_name[index] = element
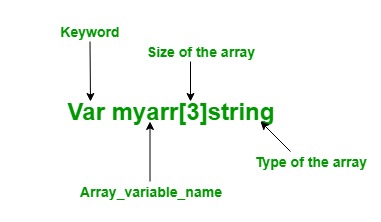
- You can access the elements of the array by using the index value or by using for loop.
- In Go language, the array type is one-dimensional.
- The length of the array is fixed and unchangeable.
- You are allowed to store duplicate elements in an array.
Approach 1: Using shorthand declaration:
In Go language, arrays can also declare using shorthand declaration. It is more flexible than the above declaration.
Syntax:
array_name:= [length]Type{item1, item2, item3,...itemN}
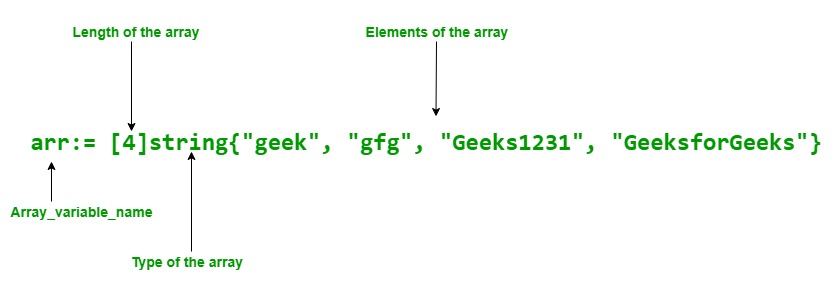
Example:
Go // Go program to illustrate how to create // an array using shorthand declaration // and accessing the elements of the // array using for loop package main import "fmt" func main() { // Shorthand declaration of array arr:= [4]string{"geek", "gfg", "Geeks1231", "GeeksforGeeks"} // Accessing the elements of // the array Using for loop fmt.Println("Elements of the array:") for i:= 0; i < 3; i++{ fmt.Println(arr[i]) } }
Output:
Elements of the array:
geek
gfg
Geeks1231
Multi-Dimensional Array
As we already know that arrays are 1-D but you are allowed to create a multi-dimensional array. Multi-Dimensional arrays are the arrays of arrays of the same type. In Go language, you can create a multi-dimensional array using the following syntax:
Array_name[Length1][Length2]..[LengthN]Type
You can create a multidimensional array using Var keyword or using shorthand declaration as shown in the below example.
Note: In a multi-dimension array, if a cell is not initialized with some value by the user, then it will initialize with zero by the compiler automatically. There is no uninitialized concept in the Golang.
Example:
Go // Go program to illustrate the // concept of multi-dimension array package main import "fmt" func main() { // Creating and initializing // 2-dimensional array // Using shorthand declaration // Here the (,) Comma is necessary arr := [3][3]string{{"C #", "C", "Python"}, {"Java", "Scala", "Perl"}, {"C++", "Go", "HTML"}} // Accessing the values of the // array Using for loop fmt.Println("Elements of Array 1") for x := 0; x < 3; x++ { for y := 0; y < 3; y++ { fmt.Println(arr[x][y]) } } // Creating a 2-dimensional // array using var keyword // and initializing a multi // -dimensional array using index var arr1 [2][2]int arr1[0][0] = 100 arr1[0][1] = 200 arr1[1][0] = 300 arr1[1][1] = 400 // Accessing the values of the array fmt.Println("Elements of array 2") for p := 0; p < 2; p++ { for q := 0; q < 2; q++ { fmt.Println(arr1[p][q]) } } }
Output:
Elements of Array 1
C#
C
Python
Java
Scala
Perl
C++
Go
HTML
Elements of array 2
100
200
300
400
Important Observations About Array
In an array, if an array does not initialize explicitly, then the default value of this array is 0.
Example:
Go // Go program to illustrate an array package main import "fmt" func main() { // Creating an array of int type // which stores two elements // Here, we do not initialize the // array so the value of the array // is zero var myarr [2]int // Printing the array fmt.Println("Elements of the Array: ", myarr) }
Output:
Elements of the Array : [0 0]
In an array, you can find the length of the array using len() method as shown below:
Example:
Go // Go program to illustrate how to find // the length of the array package main import "fmt" func main() { // Creating array // Using shorthand declaration arr1:= [3]int{9,7,6} arr2:= [...]int{9,7,6,4,5,3,2,4} arr3:= [3]int{9,3,5} // Finding the length of the // array using len method fmt.Println("Length of the array 1 is:", len(arr1)) fmt.Println("Length of the array 2 is:", len(arr2)) fmt.Println("Length of the array 3 is:", len(arr3)) }
Output:
Length of the array 1 is: 3
Length of the array 2 is: 8
Length of the array 3 is: 3
In an array, if ellipsis ‘‘...’’ become visible at the place of length, then the length of the array is determined by the initialized elements. As shown in the below example:
Example:
Go // Go program to illustrate the // concept of ellipsis in an array package main import "fmt" func main() { // Creating an array whose size is determined // by the number of elements present in it // Using ellipsis myarray:= [...]string{"GFG", "gfg", "geeks", "GeeksforGeeks", "GEEK"} fmt.Println("Elements of the array: ", myarray) // Length of the array // is determine by // Using len() method fmt.Println("Length of the array is:", len(myarray)) }
Output:
Elements of the array: [GFG gfg geeks GeeksforGeeks GEEK]
Length of the array is: 5
In an array, you are allowed to iterate over the range of the elements of the array. As shown in the below example:
Example:
Go // Go program to illustrate // how to iterate the array package main import "fmt" func main() { // Creating an array whose size // is represented by the ellipsis myarray:= [...]int{29, 79, 49, 39, 20, 49, 48, 49} // Iterate array using for loop for x:=0; x < len(myarray); x++{ fmt.Printf("%d\n", myarray[x]) } }
Output:
29
79
49
39
20
49
48
49
In Go language, an array is of value type not of reference type. So when the array is assigned to a new variable, then the changes made in the new variable do not affect the original array. As shown in the below example: Example:
Go // Go program to illustrate value type array package main import "fmt" func main() { // Creating an array whose size // is represented by the ellipsis my_array:= [...]int{100, 200, 300, 400, 500} fmt.Println("Original array(Before):", my_array) // Creating a new variable // and initialize with my_array new_array := my_array fmt.Println("New array(before):", new_array) // Change the value at index 0 to 500 new_array[0] = 500 fmt.Println("New array(After):", new_array) fmt.Println("Original array(After):", my_array) }
Output:
Original array(Before): [100 200 300 400 500]
New array(before): [100 200 300 400 500]
New array(After): [500 200 300 400 500]
Original array(After): [100 200 300 400 500]
In an array, if the element type of the array is comparable, then the array type is also comparable. So we can directly compare two arrays using == operator. As shown in the below example:
Example:
Go // Go program to illustrate // how to compare two arrays package main import "fmt" func main() { // Arrays arr1:= [3]int{9,7,6} arr2:= [...]int{9,7,6} arr3:= [3]int{9,5,3} // Comparing arrays using == operator fmt.Println(arr1==arr2) fmt.Println(arr2==arr3) fmt.Println(arr1==arr3) // This will give and error because the // type of arr1 and arr4 is a mismatch /* arr4:= [4]int{9,7,6} fmt.Println(arr1==arr4) */ }
Output:
true
false
false
Similar Reads
Go Tutorial Go or you say Golang is a procedural and statically typed programming language having the syntax similar to C programming language. It was developed in 2007 by Robert Griesemer, Rob Pike, and Ken Thompson at Google but launched in 2009 as an open-source programming language and mainly used in Google
2 min read
Overview
Go Programming Language (Introduction)Go is a procedural programming language. It was developed in 2007 by Robert Griesemer, Rob Pike, and Ken Thompson at Google but launched in 2009 as an open-source programming language. Programs are assembled by using packages, for efficient management of dependencies. This language also supports env
11 min read
How to Install Go on Windows?Prerequisite: Introduction to Go Programming Language Before, we start with the process of Installing Golang on our System. We must have first-hand knowledge of What the Go Language is and what it actually does? Go is an open-source and statically typed programming language developed in 2007 by Robe
3 min read
How to Install Golang on MacOS?Before, we start with the process of Installing Golang on our System. We must have first-hand knowledge of What the Go Language is and what it actually does? Go is an open-source and statically typed programming language developed in 2007 by Robert Griesemer, Rob Pike, and Ken Thompson at Google but
4 min read
Hello World in GolangHello, World! is the first basic program in any programming language. Letâs write the first program in the Go Language using the following steps:First of all open Go compiler. In Go language, the program is saved with .go extension and it is a UTF-8 text file.Now, first add the package main in your
3 min read
Fundamentals
Identifiers in Go LanguageIn programming languages, identifiers are used for identification purposes. In other words, identifiers are the user-defined names of the program components. In the Go language, an identifier can be a variable name, function name, constant, statement label, package name, or type. Example: package ma
3 min read
Go KeywordsKeywords or Reserved words are the words in a language that are used for some internal process or represent some predefined actions. These words are therefore not allowed to use as an identifier. Doing this will result in a compile-time error. Example: C // Go program to illustrate the // use of key
2 min read
Data Types in GoData types specify the type of data that a valid Go variable can hold. In Go language, the type is divided into four categories which are as follows: Basic type: Numbers, strings, and booleans come under this category.Aggregate type: Array and structs come under this category.Reference type: Pointer
7 min read
Go VariablesA typical program uses various values that may change during its execution. For Example, a program that performs some operations on the values entered by the user. The values entered by one user may differ from those entered by another user. Hence this makes it necessary to use variables as another
9 min read
Constants- Go LanguageAs the name CONSTANTS suggests, it means fixed. In programming languages also it is same i.e., once the value of constant is defined, it cannot be modified further. There can be any basic data type of constants like an integer constant, a floating constant, a character constant, or a string literal.
6 min read
Go OperatorsOperators are the foundation of any programming language. Thus the functionality of the Go language is incomplete without the use of operators. Operators allow us to perform different kinds of operations on operands. In the Go language, operators Can be categorized based on their different functiona
9 min read
Control Statements
Functions & Methods
Functions in Go LanguageIn Go, functions are blocks of code that perform specific tasks, which can be reused throughout the program to save memory, improve readability, and save time. Functions may or may not return a value to the caller.Example:Gopackage main import "fmt" // multiply() multiplies two integers and returns
3 min read
Variadic Functions in GoVariadic functions in Go allow you to pass a variable number of arguments to a function. This feature is useful when you donât know beforehand how many arguments you will pass. A variadic function accepts multiple arguments of the same type and can be called with any number of arguments, including n
3 min read
Anonymous function in Go LanguageAn anonymous function is a function that doesnât have a name. It is useful when you want to create an inline function. In Go, an anonymous function can also form a closure. An anonymous function is also known as a function literal.ExampleGopackage main import "fmt" func main() { // Anonymous functio
2 min read
main and init function in GolangThe Go language reserve two functions for special purpose and the functions are main() and init() function.main() functionIn Go language, the main package is a special package which is used with the programs that are executable and this package contains main() function. The main() function is a spec
2 min read
What is Blank Identifier(underscore) in Golang?_(underscore) in Golang is known as the Blank Identifier. Identifiers are the user-defined name of the program components used for the identification purpose. Golang has a special feature to define and use the unused variable using Blank Identifier. Unused variables are those variables that are defi
3 min read
Defer Keyword in GolangIn Go language, defer statements delay the execution of the function or method or an anonymous method until the nearby functions returns. In other words, defer function or method call arguments evaluate instantly, but they don't execute until the nearby functions returns. You can create a deferred m
3 min read
Methods in GolangGo methods are like functions but with a key difference: they have a receiver argument, which allows access to the receiver's properties. The receiver can be a struct or non-struct type, but both must be in the same package. Methods cannot be created for types defined in other packages, including bu
3 min read
Structure
Arrays
Slices
Slices in GolangSlices in Go are a flexible and efficient way to represent arrays, and they are often used in place of arrays because of their dynamic size and added features. A slice is a reference to a portion of an array. It's a data structure that describes a portion of an array by specifying the starting index
14 min read
Slice Composite Literal in GoThere are two terms i.e. Slice and Composite Literal. Slice is a composite data type similar to an array which is used to hold the elements of the same data type. The main difference between array and slice is that slice can vary in size dynamically but not an array. Composite literals are used to c
3 min read
How to sort a slice of ints in Golang?In Go, slices provide a flexible way to manage sequences of elements. To sort a slice of ints, the sort package offers a few straightforward functions. In this article we will learn How to Sort a Slice of Ints in Golang.ExampleGopackage main import ( "fmt" "sort" ) func main() { intSlice := []int{42
2 min read
How to trim a slice of bytes in Golang?In Go language slice is more powerful, flexible, convenient than an array, and is a lightweight data structure. The slice is a variable-length sequence which stores elements of a similar type, you are not allowed to store different type of elements in the same slice. In the Go slice of bytes, you ar
3 min read
How to split a slice of bytes in Golang?In Golang, you can split a slice of bytes into multiple parts using the bytes.Split function. This is useful when dealing with data like encoded strings, file contents, or byte streams that must be divided by a specific delimiter.Examplepackage mainimport ( "bytes" "fmt")func main() { // Initial byt
3 min read
Strings
Strings in GolangIn the Go language, strings are different from other languages like Java, C++, Python, etc. It is a sequence of variable-width characters where every character is represented by one or more bytes using UTF-8 Encoding. In other words, strings are the immutable chain of arbitrary bytes(including bytes
7 min read
How to Trim a String in Golang?In Go, strings are UTF-8 encoded sequences of variable-width characters, unlike some other languages like Java, python and C++. Go provides several functions within the strings package to trim characters from strings.In this article we will learn how to Trim a String in Golang.Examples := "@@Hello,
2 min read
How to Split a String in Golang?In Go language, strings differ from other languages like Java, C++, and Python. A string in Go is a sequence of variable-width characters, with each character represented by one or more bytes using UTF-8 encoding. In Go, you can split a string into a slice using several functions provided in the str
3 min read
Different ways to compare Strings in GolangIn Go, strings are immutable sequences of bytes encoded in UTF-8. You can compare them using comparison operators or the strings.Compare function. In this article,we will learn different ways to compare Strings in Golang.Examplepackage main import ( "fmt" "strings" ) func main() { s1 := "Hello" s2 :
2 min read
Pointers