Android | Display Multiplication Table of a Number
Last Updated : 30 Apr, 2024
Given a number, the task is to display the multiplication table of this number using the Android App.
Steps to Build an Android Application for Multiplication Table
We will follow the Steps in the order they are mentioned below to create the application.
Step 1: Create a new Android Application and Name it Program_Multiplication_Table with the Empty layout.
Note: We have used the name of the Program_Multiplication_Table as the Name of the Application. Please change the package name if you are using different name.
Step 2: Open the activity_main.xml (values>layout>activity_main.xml) file where we will be creating the layout of the application.
Step 3: In activity_main.xml file add TextView, EditText, and a Button.
The Component Tree will look like this :
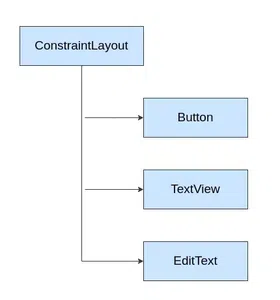
Step 4: Assign ID to each component
Step 5: Now, open up the MainActivity file and declare the variables.
Step 6: Read the values entered in the EditText boxes using an ID that has been set in the XML code above.
Step 7: Add a click listener to the Add button
Step 8: When the Add button has been clicked we need to Multiply the values and store it in Buffer
Step 9: Then show the resultant output in the TextView by setting the buffer in the TextView.
Implementation to Build Display Multiplication Table of a Number
Below are the codes for MainActivity.java and activity_main.xml to build android application which displays the multiplication table of a number:
MainActivity.java // Build the java logic for multiplication table // using button, text view, edit text package com.example.program_multiplication_table; import androidx.appcompat.app.AppCompatActivity; import android.os.Bundle; import android.view.View; import android.widget.Button; import android.widget.EditText; import android.widget.TextView; public class MainActivity extends AppCompatActivity implements View.OnClickListener { // define the global variable // variable number1, number2 for input input number // Add_button, result textView EditText editText; Button button; TextView result; int ans = 0; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); // by ID we can use each component // whose id is assigned in the XML file editText = (EditText)findViewById(R.id.editText); button = (Button)findViewById(R.id.button); result = (TextView)findViewById(R.id.textView); // set clickListener on button button.setOnClickListener(this); } @Override public void onClick(View v) { if(v.getId()==R.id.button) { StringBuffer buffer = new StringBuffer(); // get the input number from editText String fs = editText.getText().toString(); // convert it to integer int n = Integer.parseInt(fs); // build the logic for table for (int i = 1; i <= 10; i++) { ans = (i * n); buffer.append(n + " X " + i + " = " + ans + "\n\n"); } // set the buffer textview result.setText(buffer); } } }
activity_main.xml <?xml version="1.0" encoding="utf-8"?> <androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity" tools:layout_editor_absoluteY="25dp"> <!-- Add the button for run table logic and print result--> <!-- give id "button"--> <Button android:id="@+id/button" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginTop="16dp" android:text="TABLE" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintStart_toEndOf="@+id/editText" app:layout_constraintTop_toTopOf="parent" /> <!-- Text view for result view--> <!-- give the id TextView--> <TextView android:id="@+id/textView" android:layout_width="0dp" android:layout_height="0dp" android:layout_marginStart="36dp" android:layout_marginEnd="36dp" android:layout_marginBottom="18dp" android:textColor="@color/black" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toBottomOf="@+id/editText" /> <!-- edit Text for take input from user--> <!-- give the id editText--> <EditText android:id="@+id/editText" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginBottom="20dp" android:layout_marginEnd="9dp" android:layout_marginRight="9dp" android:layout_marginTop="16dp" android:ems="10" android:inputType="number" app:layout_constraintBottom_toTopOf="@+id/textView2" app:layout_constraintEnd_toStartOf="@+id/button" app:layout_constraintHorizontal_chainStyle="packed" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toTopOf="parent" tools:ignore="UnknownId" /> </androidx.constraintlayout.widget.ConstraintLayout>
Application Look:
Similar Reads
Android Application to Add Two Numbers
Below are the steps for Creating a Simple Android Application to Add Two Numbers Note: Similarly, Android App to subtract, multiply and divide numbers can be made by making minor changes in the Java and XML code. Step-by-Step Implementation of Application to Add Two Numbers in AndroidStep 1: Opening
3 min read
Implement Phone Number Validator in Android
Phone validation is useful for users to check whether the number entered by the user is a valid number or not. If the user has entered the number is not valid then it will show a Toast message to re-enter the number. In this article, we are going to discuss how to implement the phone number validato
3 min read
How to Build a Number Shapes Android App in Android Studio?
Android is a vast field to learn and explore and to make this learning journey more interesting and productive we should keep trying new problems with new logic. So, today we are going to develop an application in android studio which tells us about Numbers that have shapes. We will be covering two
4 min read
Build an Android App to Check a Number is Armstrong or Not
What is Armstrong's number? A number is said to be Armstrong if the sum of its digits is raised to the power of the total number of digits in the number. Given a number x, determine whether the given number is Armstrong's number or not. A positive integer of n digits is called an Armstrong number of
3 min read
Build an Android App to Check a Number is Automorphic or Not
What is an Automorphic number? A number is said to be Automorphic if its square ends in the same digits as the number itself. Examples: Input : N = 76 Output : Automorphic Explanation: As 76*76 = 5776 Input : N = 25 Output : Automorphic As 25*25 = 625 Input : N = 7 Output : Not Automorphic As 7*7 =
3 min read
How to Build a Prime Number Checker Android App in Android Studio?
A prime number is a natural number greater than 1, which is only divisible by 1 and itself. First few prime numbers are : 2 3 5 7 11 13 17 19 23 â¦.. . In this article, we will be building a Prime Number Checker android app in Android Studio using Kotlin and XML. The app will check whether the entere
3 min read
How to Display Percentage on a ProgressBar in Android?
Android ProgressBar is a visual representation or graphical view, that is used to indicate the progress of an operation or task. Android progress bar can be used to show any numeric value. There are many types of progress bars: Spinning Wheel ProgressBarHorizontal ProgressBar In the given article we
3 min read
Date and Time Formatting in Android
Date and Time in Android are formatted using the SimpleDateFormat library from Java, using Calendar instance which helps to get the current system date and time. The current date and time are of the type Long which can be converted to a human-readable date and time. In this article, it's been discus
5 min read
Guessing the Number Game using Android Studio
A Simple Guess the number application in which application generates the random number between 1 to 100 and the user has to guess that Number. It is a simple and basic application that uses basic widgets and libraries. Approach: Step1: Creating a new project Click on File option at topmost corner in
3 min read
How to Build a Cryptocurrency Tracker Android App?
CryptoCurrency nowadays is in most demand and many people are investing in these currencies to get high returns. Many websites and applications provide us information about the rates of different cryptocurrencies available in the Crypto Market. In this article, we will be building a similar applicat
10 min read